#라이브러리 불러오기
import matplotlib.pyplot as plt
import seaborn as sns
import pandas as pd
import numpy as np
tips = sns. load_dataset ("tips")
fig, ax =plt.subplots() #그래프 틀
ax.plot([1, 2, 3]) #그래프 속의 수치
sns.scatterplot(x = 'total_bill', y= 'tip', hue = 'sex', ax = ax, data= tips)
##plt.savefig("output/result.png") #output 폴더에 png 로 저장 (주피터랩)
plt.show()
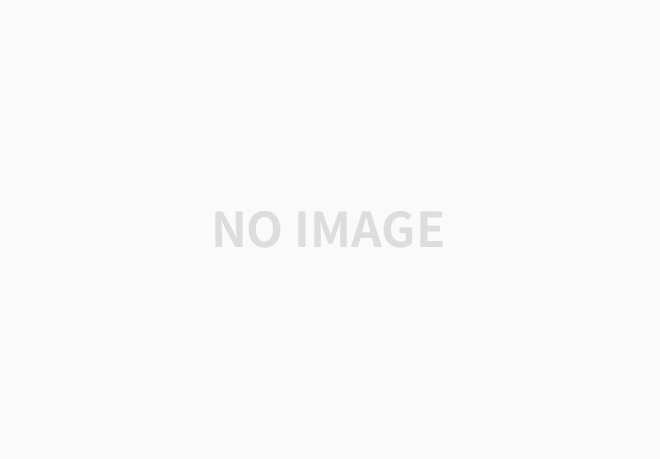
#matplot lib seaborn 동시에 사용subplotting 기법
fig,ax = plt.subplots(nrows =1,ncols= 2) #nrows = number of rows ncols = number of columns 1행 2열로 생성한다
plt.title("plt.title") # 타이틀이 오른족 그래프에 가서 붙는다
plt.show
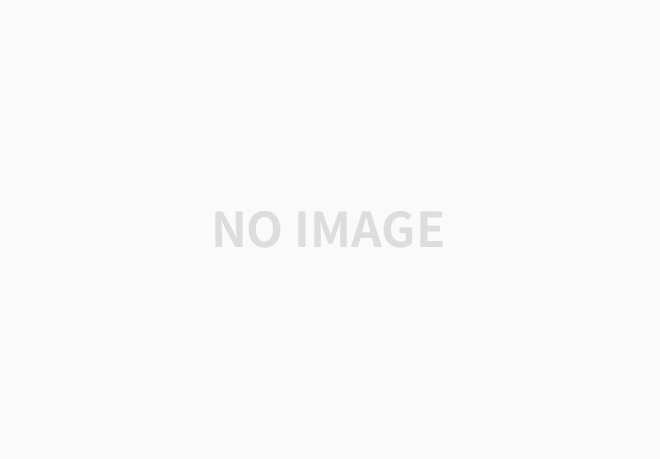
fig,ax = plt.subplots(nrows =2,ncols= 2) # 2행 2열
ax[0,0].set_title("ax[0] title")
ax[0,0].plot ([1,2,3])
ax[0,1].set_title("ax[0] title")
ax[0,1].plot ([1,2,3])
ax[1,0].set_title("ax[1] title")
sns.scatterplot(x = 'total_bill', y= 'tip', hue = 'sex', ax = ax[1,0], data= tips)#요소 와 위치 모두 지정해줘야함
ax[1,1].set_title("ax[1] title")
sns.scatterplot(x = 'total_bill', y= 'tip', hue = 'sex', ax = ax[1,1], data= tips)
fig.suptitle("fig.title") #전체 그래프 타이틀
plt.tight_layout()
plt.show
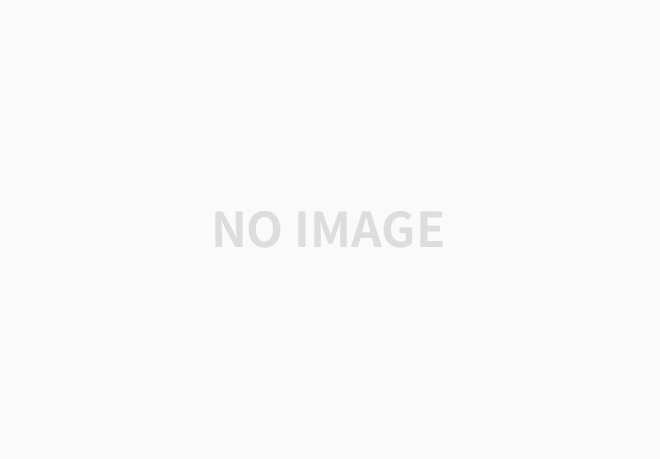
#산점도 그래프
- x축 y축 데이터형태가 연속형 데이터가 와야함
- 연속형 데이터의 예시 : 키 몸무게등 소수점이 존재하는데이터
fig, ax=plt.subplots(1, 2, figsize=(15, 5))
sns.regplot(x="total_bill", y="tip", data=tips, ax=ax[0], fit_reg=True) #fitreg = 선
ax[0].set_title('with linear regression line')
sns.regplot(x="total_bill", y="tip", data=tips, ax=ax[1], fit_reg=False)
ax[1].set_title('without linear regression line')
plt.show()
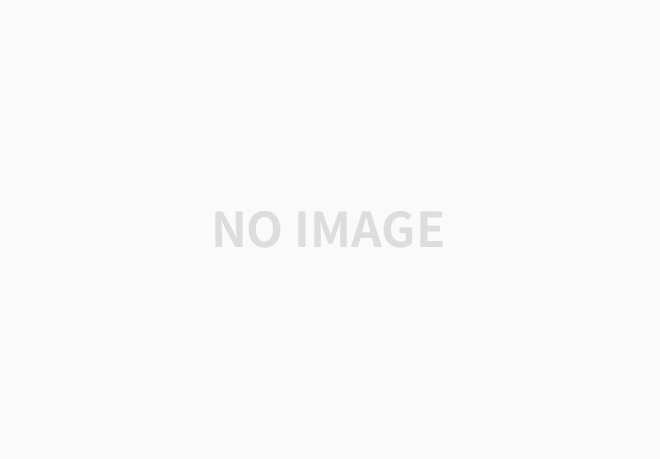
fig,ax = plt.subplots()
sns.regplot(x="day", y="tip", data=tips, ax=ax, fit_reg=False)
ax.set_title('without linear regression line')
plt.show()
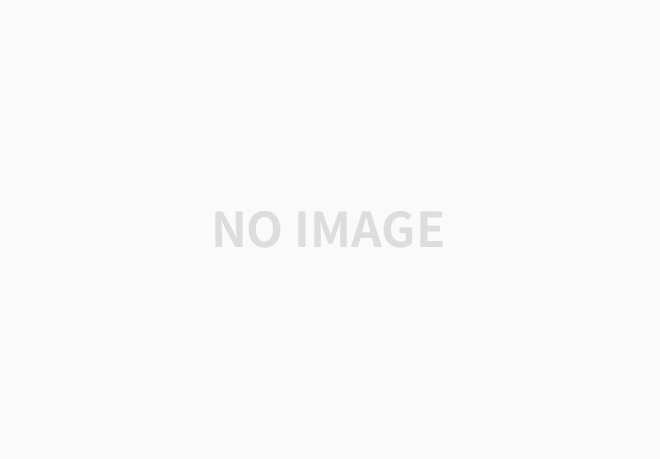
fig, ax = plt.subplots()
#sns.distplot(x= 'tip',ax= ax, data=tips) 에러메시지만 확인 ax미존재
sns.histplot(x= 'tip',ax= ax, data=tips) #히스플롯 히스토그램
plt.show()
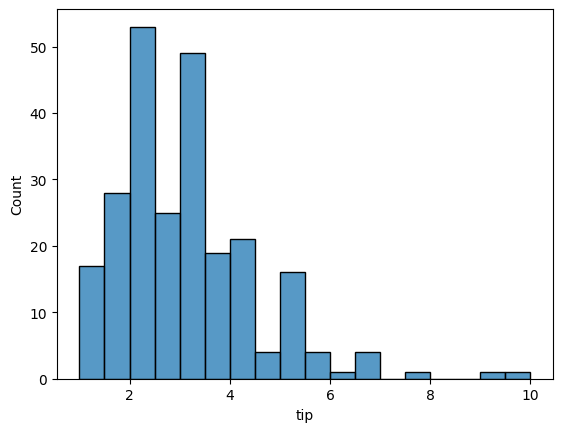
fig, ax = plt.subplots(nrows = 1, ncols = 2)
sns.boxplot(x = 'sex', y = 'tip', ax = ax[0], data = tips)
sns.swarmplot(x = 'sex', y = 'tip', ax = ax[0], data = tips, alpha= 0.5)
sns.scatterplot(x = 'total_bill', y = 'tip', hue = 'sex', ax = ax[1], data = tips)
plt.show()
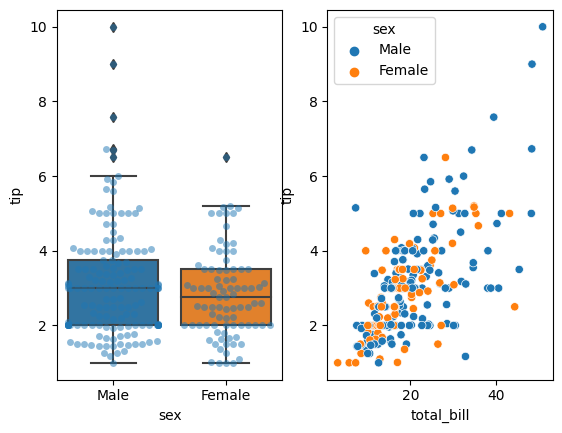
sns.countplot(x = 'day',data=tips)
plt.show()
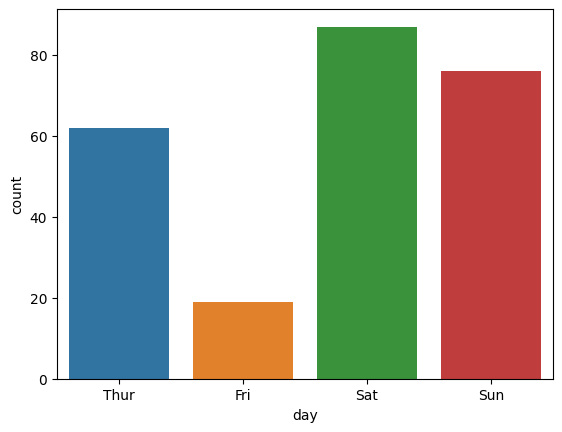
sns.countplot(x = 'day',data=tips, order = tips['day'].value_counts().index)
plt.show()
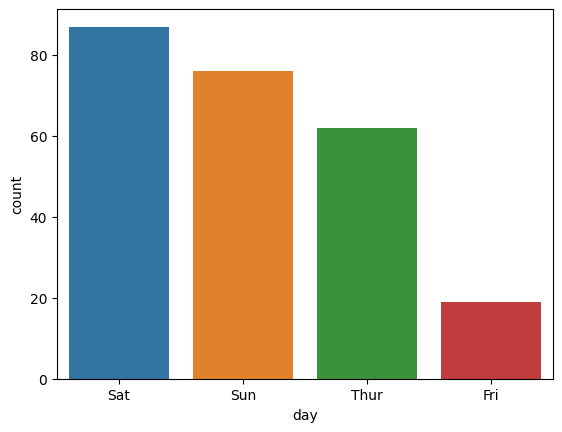
import matplotlib.pyplot as plt
from matplotlib.ticker import (MultipleLocator, AutoMinorLocator, FuncFormatter) #축설정 할때 값
import seaborn as sns
import numpy as np
#y 축 설정할때 값을 소수점 2자리까지 표현
def major_formatter(x, pos):
return "%.2f$" % x
formatter = FuncFormatter(major_formatter)
fig, ax = plt.subplots(nrows = 1, ncols = 2, figsize=(16, 5))
# 이상적인 막대 그래프
ax0 = sns.barplot(x = "day", y = 'total_bill', data = tips,
errorbar=None, color='lightgray', zorder=2, alpha=0.85, ax=ax[0]) # zorder=2,
group_mean = tips.groupby(['day'])['total_bill'].agg('mean')
# print(group_mean)
h_day = group_mean.sort_values(ascending=False).index[0] # 해당되는 결괏값의 MAX값의 인덱스 요일을 하나 가져옴
h_mean = np.round(group_mean.sort_values(ascending=False)[0], 2) # 해당되는 결괏값의 MAX값을 하나 가져옴
for p in ax0.patches:
print(type(p))
fontweight = "normal"
color = "k"
height = np.round(p.get_height(), 2)
print(height)
if h_mean == height:
fontweight="bold"
color="darkred" # Adobe Color Wheel 색상코드 추가
p.set_facecolor(color)
p.set_edgecolor("black")
ax0.text(p.get_x() + p.get_width()/2., height+1, height, ha = 'center',
size=12,
fontweight=fontweight, color=color)
# y축 범위 지정 옵션
ax0.set_ylim(-3, 30)
ax0.set_title("Ideal Bar Graph", size = 16)
# 가로세로 축, 일부 수정 및 변경
ax0.spines['top'].set_visible(False)
ax0.spines['left'].set_position(("outward", 20))
ax0.spines['left'].set_visible(False)
ax0.spines['right'].set_visible(False)
# y축 값 변경
ax0.yaxis.set_major_locator(MultipleLocator(10))
ax0.yaxis.set_major_formatter(formatter)
ax0.yaxis.set_minor_locator(MultipleLocator(5))
# y축 라벨 변경
ax0.set_ylabel("Avg. Total Bill($)", fontsize=14)
# y축 Grid 라인 수정
ax0.grid(axis="y", which="major", color="lightgray")
ax0.grid(axis="y", which="minor", ls=":")
# x축의 값을 변경
ax0.set_xlabel("Weekday", fontsize=14)
for xtick in ax0.get_xticklabels():
print(xtick)
if xtick.get_text() == h_day:
xtick.set_color("darkred")
xtick.set_fontweight("demibold")
ax0.set_xticklabels(['Thursday', 'Friday', 'Saturday', 'Sunday'], size=12)
# 그냥 일반 막대 그래프
ax1 = sns.barplot(x = "day", y = 'total_bill', data = tips, errorbar=None, alpha=0.85, ax=ax[1])
for p in ax1.patches:
height = np.round(p.get_height(), 2)
ax1.text(p.get_x() + p.get_width()/2., height+1, height, ha = 'center', size=12)
ax1.set_ylim(-3, 30)
ax1.set_title("Just Bar Graph")
plt.show()
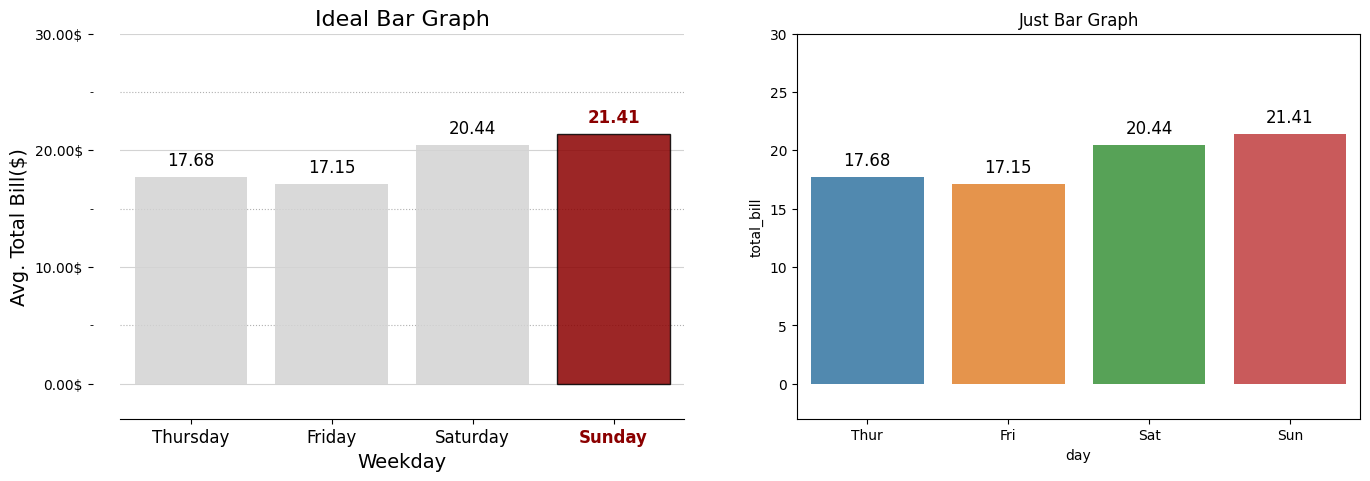
'수업외 정리' 카테고리의 다른 글
24-01-11 통계실습 통계검정 (0) | 2024.01.11 |
---|---|
24-01-10 통계 (0) | 2024.01.11 |
240108복습 데이터 불러오기 (1) | 2024.01.09 |
240108복습 데이터 통합하기 (0) | 2024.01.08 |
240108복습 merge (0) | 2024.01.08 |